Introduction
A cross-platform app that can function seamlessly on any platform or device without facing operational, security, or layout issues sounds implausible until React Native takes the reins.
This famous JavaScript-based mobile app framework permits you to render mobile apps for both iOS and Android natively.
The framework has the same surfboard (codebase) that can be used effectively on different waters (platforms).
A major credit for building mobile apps through React Native goes to the testing department of the project.
Since testing is significant to develop a smooth functioning app, it is also important to make sure that the app is unit tested efficiently and thoroughly.
Rigorous testing helps eliminate bugs and other issues in the app, delivering a smooth user experience.
Hence, testing is an integral part of developing a mobile app through React.
This article will focus on the different testing challenges, strategies, and ways to overcome React Native development issues arising in the code.
Key Components of React Native Testing
Before tackling the multifarious hassles that developers face in React, let us first understand the different components that build up such rigorous testing during the app development process. These components are mentioned below:
Unit Testing
Unit testing evaluates the different components of a mobile application at an individual level. This is done to analyze the upsides and shortcomings of instilling an individual component of the mobile application.
A component is considered to be the most basic part of an app. If a component fails the test, it is not necessarily a bad thing.
Think of it as something that hinders your app from properly functioning and needs working on so that your app can function properly.
At least, this way you can discern the working components from the buggy ones.
Significance of Unit Testing
Unit testing does not help out in a single category but multiple. Some of the salient reasons why it is important are as follows:
- Quicker Development- Bugs in general, can be detected early on through such a process. Detecting such bugs before the production or release stage and eliminating them saves a lot of time and money on the client side.
-
- Enhanced Code Quality- Rigorous testing refines the code’s quality and provides insights consistently as to things that can be improved. The code becomes loosely coupled and more modular through such testing simultaneously improving the code quality.
- Enhanced Code Quality- Rigorous testing refines the code’s quality and provides insights consistently as to things that can be improved. The code becomes loosely coupled and more modular through such testing simultaneously improving the code quality.
-
- Code Reliability and Maintenance- Reliable and understandable code can be attained through unit testing that can resolve bugs in the program early on. Maintaining such a code can be done instantly by anyone who has unit-tested the application multiple times.
- Code Reliability and Maintenance- Reliable and understandable code can be attained through unit testing that can resolve bugs in the program early on. Maintaining such a code can be done instantly by anyone who has unit-tested the application multiple times.
-
- Better documentation- Unit Testing helps in organized documentation of your code which assists other developers in interpreting the way your code works. Such documentation helps out other developers when they take over your project, making things easier for them.
- Better documentation- Unit Testing helps in organized documentation of your code which assists other developers in interpreting the way your code works. Such documentation helps out other developers when they take over your project, making things easier for them.
Some of the libraries and frameworks that can help you unit test components individually are Jasmine, Enzyme, Jest, and React Testing Library.
Integration Testing
For a more holistic type of testing, integration testing comes in handy.
The entire system is tested here instead of individual components to evaluate if multiple components interact seamlessly with each other to ensure they work as intended.
They validate the interactions between different modules or components of a system, analyzing if the integrated parts work well together.
Some of the testing frameworks or libraries that can be used for Integration Testing are Detox and React Native Testing Library.
End-to-End (E2E) Testing
This kind of testing involves the complete workflow of the app generally from a user’s perspective to make sure all the parts work completely fine which includes UI as well as navigation.
By utilizing user case scenarios, it aims to confirm that the entire process goes smoothly and that its user experience and functionality work effectively.
Some of the tools that can help out during End-to-end testing of the mobile app are Detox, Appium, and Maestro. The latter two are utilized for iOS and Android app development distinctively.
There are two kinds of E2E testing- Vertical and Horizontal testing.
Vertical tests performed in chronological order are utilized in examining complex computing systems.
Horizontal testing, on the other hand, is done considering the user’s needs and perspective keeping in mind that the app functions perfectly from the user’s side.
There are two kinds of E2E testing- Vertical and Horizontal testing.
Vertical: Verticle Tests performed in chronological order are utilized in examining complex computing systems.
Horizontal: Horizontal testing, on the other hand, is done considering the user’s needs and perspective keeping in mind that the app functions perfectly from the user’s side.
Common Challenges in React Native Testing
Now that you are aware of the key testing methods and components required for the smooth functioning of your mobile application, it is time to shed some light on a few challenges faced during React Native Testing. Some of these challenges are:
Device Fragmentation
One of the sternest and loftiest challenges to overcome is the issue of device fragmentation.
It accounts for the inconsistency of iOS and Android versions in different devices that a user faces.
This can fairly complicate things to develop an app that functions smoothly and efficiently in different versions of a device.
It has always been a hassle to overcome device fragmentation in React Native. To facilitate cross-platform app development, there are many React Native app testing strategies you can adopt in your program.
The impact these strategies make will depend on the screen size of the device, the operating system version, and the aspect ratio of the device.
-
- For a seamless user experience, developers must embody responsive design strategies and completely test the app across multiple devices.
- For a seamless user experience, developers must embody responsive design strategies and completely test the app across multiple devices.
-
- It is important to consider cutting-edge security measures to cease device fragmentation from taking anything away from the safety of the user’s information.
- It is important to consider cutting-edge security measures to cease device fragmentation from taking anything away from the safety of the user’s information.
-
- Developers need to implement safe coding practices and incorporate suitable methods for authentication and authorization to safeguard their data.
- Developers need to implement safe coding practices and incorporate suitable methods for authentication and authorization to safeguard their data.
-
- Make sure to conduct frequent security audits for curtailed security risks and remain consistent with the best security methods.
Asynchronous Operations
Asynchronous code implies that you can run some parts of your code while the remaining parts are still awaiting further instructions and data.
This makes things easier for the developers as they no longer have to wait to execute the important parts of the app until the less important ones are rendered.
This code also helps in updating the application by displaying and requesting new information offering a seamless user experience even during the development of long functions and requests.
It can be a hassle to manage asynchronous code for the mobile app in React Native. Some of the strategies that might help in these operations are:
-
- Testing Frameworks– The only frameworks to utilize for better management of the asynchronous code are the ones supporting asynchronous testing. Some of these might be Jest for JavaScript and PyTest for Python.
- Testing Frameworks– The only frameworks to utilize for better management of the asynchronous code are the ones supporting asynchronous testing. Some of these might be Jest for JavaScript and PyTest for Python.
-
- Mocking– Mocks and stubs help out a lot for asynchronous dependencies like APIs, database management software,or external services. This ensures the isolation and predictability of your tests.
- Mocking– Mocks and stubs help out a lot for asynchronous dependencies like APIs, database management software,or external services. This ensures the isolation and predictability of your tests.
-
- Promises and Callbacks– Async operations can also be managed through languages that support async/await or Promises. This makes your code simpler to read.
- Promises and Callbacks– Async operations can also be managed through languages that support async/await or Promises. This makes your code simpler to read.
-
- Timeouts– Setting accurate timeouts for async testing makes things much easier. Longer timeouts might defer test runs whereas shorter ones would deliver false negatives. Hence, make sure to keep them adequate.
- Timeouts– Setting accurate timeouts for async testing makes things much easier. Longer timeouts might defer test runs whereas shorter ones would deliver false negatives. Hence, make sure to keep them adequate.
Testing Native Modules
One of the greatest things about React Native is that it supports all kinds of modules and APIs. This enhances the development speed of the project.
However, not all problems can be remedied through React Native modules. When it comes to niche and specific problems, React Native deals with an impasse.
Additionally, if the native components are available to the developer, the updated version of the OS might not be available.
However, this problem can be solved by building a part of the app with native technologies.
If your problem is quite specific and unique, hindering you from finding a solution to the problem, you can develop your custom module.
Such solutions can help you overcome the challenges faced during native module testing.
Since it is all about finding the remedy to a unique and niche problem, it is much better to develop native solutions to a native problem.
React Native can help you out in other departments.
Best Practices to Overcome Testing Challenges
Since React Native deals with a lot of challenges in its framework, it is time to understand your way out of these issues. Below is a list of strategies you can utilize to overcome these testing challenges:
TDD in React Native
It is important to understand what Test-Driven Development implies. It is an approach that focuses on writing tests on paper before you can begin to write actual code.
Test cases assist developers in spotting the faulty lines in their code determining if it meets the user’s requirements or not.
TDD rigorously tests the code from the beginning scrutinizing any shortcomings in the program.
In a way, it not only delivers enhanced code quality but also the development process and encourages thoughtful design.
CI/CD (Continuous Integration and Deployment)
CI/CD involves frequently integrating automation testing, code changes, and application deployment.
Such a method makes sure that developers can identify bugs early on, maintain code quality, and expeditiously deliver better features and updates.
CI/CD helps developers in React Native streamline their development workflow.
CI/CD helps in rapid iteration that incentivizes developers to publish newer features along with debugging.
Such speed in solving issues aids businesses in staying competitive delivering value to their users and target audience quicker.
The CI/CD pipeline has automated testing integrated into it that can catch any bugs or issues early on mitigating the risk of coding failure.
The role of automated tests is to make sure that critical functioning isn’t jeopardized with every code update.
Mocking and Stubbing
Both Mocking and Stubbing can be used interchangeably however, they are not the same. They help in building controlled and anticipated environments for testing code but vary in their diverse approaches.
Mocking deals with developing a fake image of an object that functions exactly like the real object.
These objects validate whether the analyzed code calls the method on the mocked object and delivers the desired result or not.
Stubbing is the development of a false object or function returning the pre-determined values for analyzing certain code paths.
Without completely delivering the functionality, Stub objects simulate the operability of a real object.
Both these techniques are utilized to ensure that all the elements and components of the code run smoothly and efficiently.
These techniques are used in React Native to mimic external components or services to focus on certain scenarios without instilling the complete production.
Future Trends in React Native Testing
While you can make changes in your code based on the issues you face following the four mentioned steps, it is also significant to understand where the market will go in a few years.
The following trends are estimated to be in vogue in the next few years:
AI Integration
As we see the innovation in the mobile app market, we can estimate more React Native apps integrating with AI and machine learning technologies.
Some of the features these technologies can offer are image recognition, language processing, and recommendation systems.
Hence, you can expect a lot more personalized and intelligent apps that offer individual suggestions for users, scale to their interaction, anticipate their expressions, and so on.
Serverless architecture and Cloud integration
Serverless architecture such as Google Cloud Functions or AWS Lambda allows developers to build cost-effective and highly scalable backend solutions.
Such a method can handle multiple workloads while limiting the server management complexity.
AR and VR Implementations
With the help of ARKit and ARCore being the only AR and VR extensions in React Native, you can enter the world of a more immersive and interactive kind.
Hence, this trend is already in motion since it has a fairly high demand at the moment.
TypeScript Adoption
TypeScript is a statically typed superset of JavaScript which has been the talk of the town in the React Native Community.
The reasons for that are the benefits it offers like improved error analysis, improved code communication, and enhanced development workflows.
Its React Native integration allows developers to write more dependable and manageable code.
Conclusion
If you made it here, it means you have gathered the fundamental knowledge about different types of testing included in React Native.
You must also have realized the challenges developers face in dealing with apps during their testing phase. It is a matter of concern that there might be quite a few bugs in one’s code.
However, with the right solutions offered and the right execution, you do not need to worry since such practices won’t betray your efforts.
React developers need to understand the loopholes that might occur in their program along with their solutions to deliver a seamlessly functioning and highly efficient mobile app to their clients.
Frequently Asked Question’s (FAQ’s)
1. How to ensure that the React Native app is compatible with the latest OS updates?
Developers frequently need to update their app’s dependencies containing third-party modules and React Native libraries to eliminate potential compatibility issues. You can also test the beta versions of the new OS releases to identify and resolve any compatibility issues in advance.
2. What is the purpose of End-To-End Testing and how does it contribute to the overall app quality?
End-to-end testing involves simulating real interactions between different components across the application. This testing approach allows spotting and eliminating user flow-related issues to offer a seamless user experience in React Native apps.
3. How can developers manage asynchronous operations in React Native testing?
JavaScript’s async nature demands developers to turn on async/await as well as promises to maintain the asynchronous code. Implementing the best practices to analyze asynchronous operations makes sure of the app’s stability in multiple conditions.
4. What steps to take to optimize the testing process for React Native apps with large codebases?
Large codebases require modularization, deploy testing strategies such as parallel test execution, and utilize tools offering code coverage analysis. Such practices offer more dependable and expeditious testing outcomes. database management software
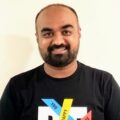
Vihar Rana
Working as a project manager in 360 Degree Technosoft since its inception. Loves to learn new technology, train the team with the latest technology advancement, develop mobile apps, and share the knowledge. I love to write on Android and iOS updates, a guide to developing apps, recent designing trends, and such subjects.